TypeScript 015 typeof YouTube

TypeScript typeof How does typeof work in TypeScript?
typeof myfruit is a value, not a type. So it's the JavaScript typeof operator, not the TypeScript type query operator. It will always return the value "string"; it will never be Fruit or "Fruit". You can't get the results of the TypeScript type query operator at runtime, because the type system is erased at runtime.

Creating a class using typescript with specific fields Typescript
TypeScript type guards help assure the value of a type, improving the overall code flow. In this article, we reviewed several of the most helpful type guards in TypeScript, exploring a few examples to see them in action. Oftentimes, your use case can be solved using either the instanceof type guard, the typeof type guard, or the in type guard.

What is Class and How to use Class in TypeScript?
Alternatively, we can directly pass the value we'd like and TypeScript will infer the type automatically using Type Inference. 1. 2. const result = identity("I love CopyCat's Figma Plugin"); console.log(typeof(result)); When you run the above function, it will return the string type as the data type of the function.
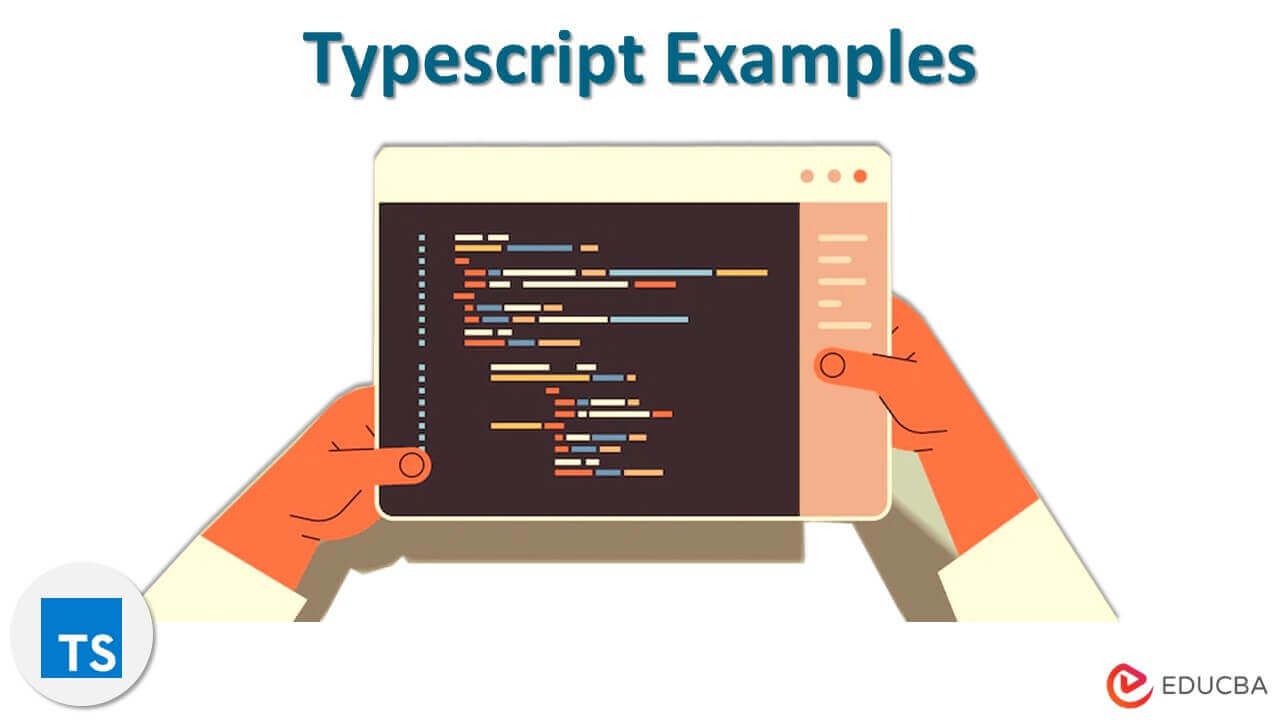
Typescript Examples All Typescript with Examples
TypeScript is a strongly typed language that is a superset of JavaScript. It offers built-in type-checking mechanisms to catch errors during web development and uses a structural type system that allows for a rich and expressive way to define and validate the shape of your objects. This improves your code readability and understanding.
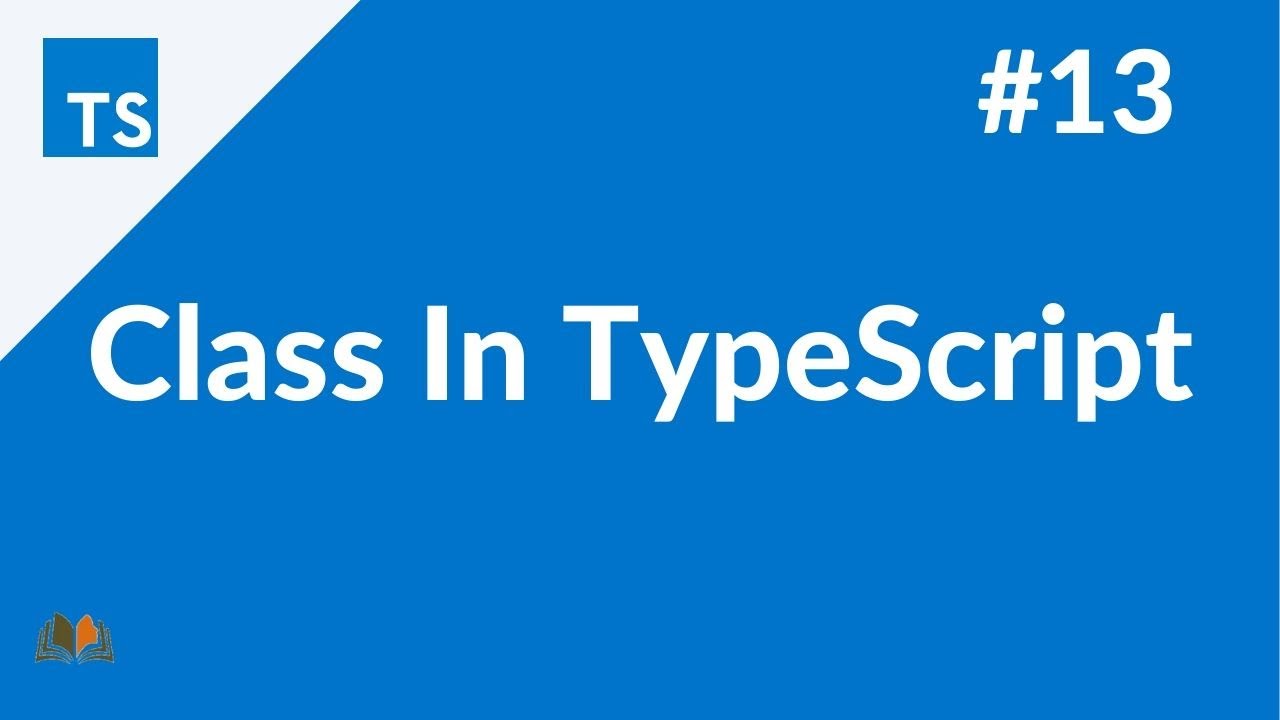
Class in TypeScript 13 TypeScript Tutorial VCreationsTech YouTube
In TypeScript, types are overlaid onto JavaScript code through an entirely separate type system, rather than becoming part of the JavaScript code itself. This means that an interface ("type") in TypeScript can—and often does—use the same identifier name as a variable in JavaScript without introducing a name conflict.

Classes (TypeScript tutorial, 7) YouTube
Classes are both a type and a value in TypeScript, and as such, can be used both ways. To use a class as a type, you use the class name in any place that TypeScript expects a type. For example, given the Employee class you created previously: class Employee { constructor( public identifier: string ) {} }
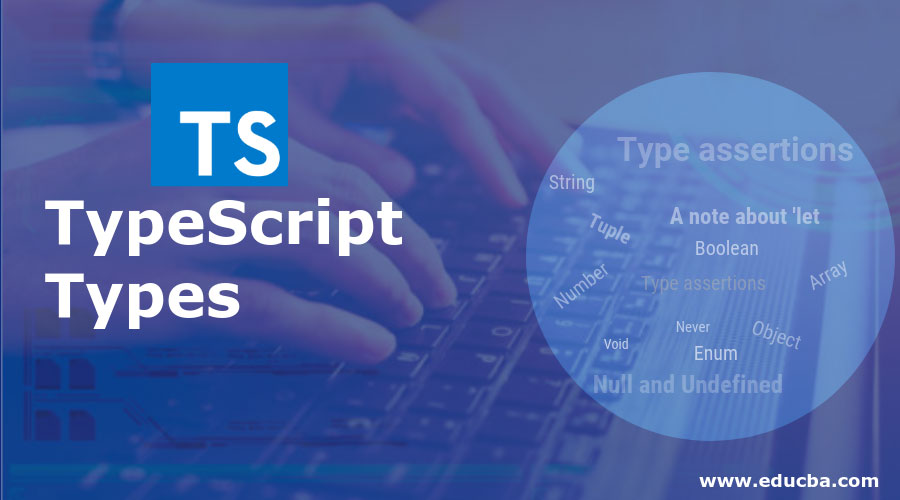
TypeScript Types 12 Amazing Types of TypeScript You Need to Know
TypeScript doesn't use "types on the left"-style declarations like int x = 0; Type annotations will always go after the thing being typed.. In most cases, though, this isn't needed. Wherever possible, TypeScript tries to automatically infer the types in your code. For example, the type of a variable is inferred based on the type of its initializer:
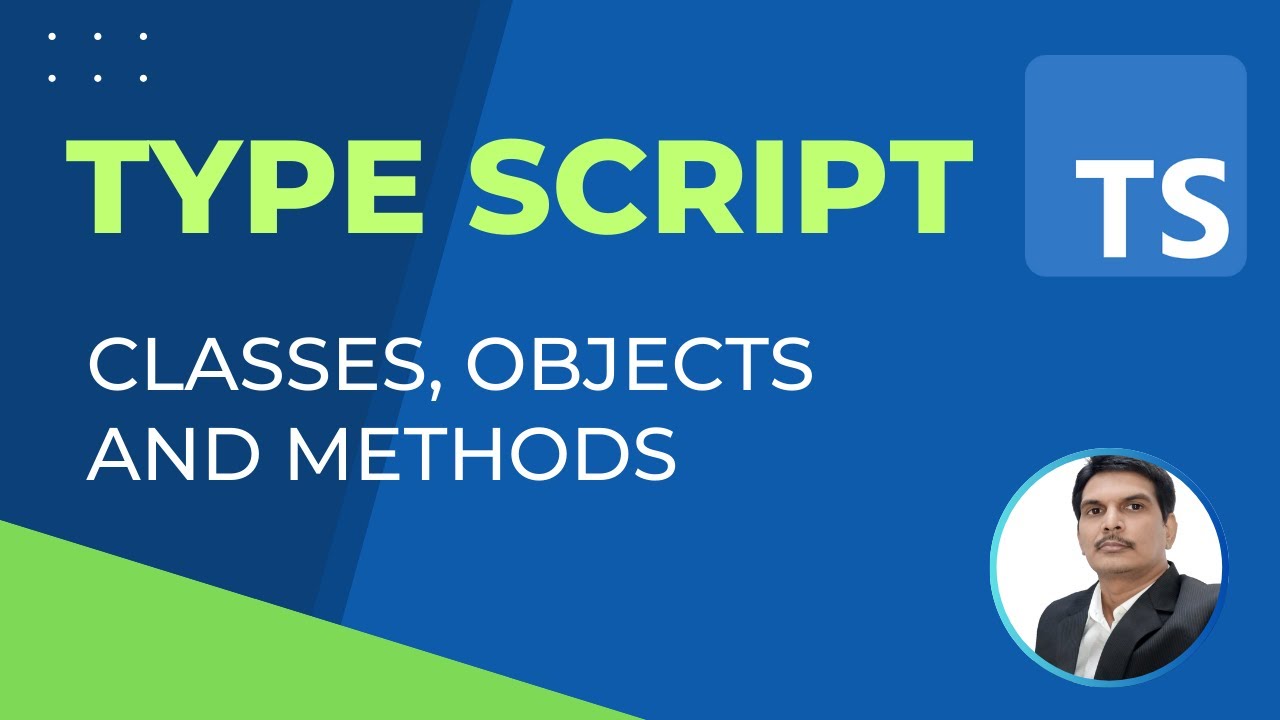
TypeScript Part12 Classes Objects Methods YouTube
48. You could use TypeScript's built in InstanceType for that: private delegate: InstanceType
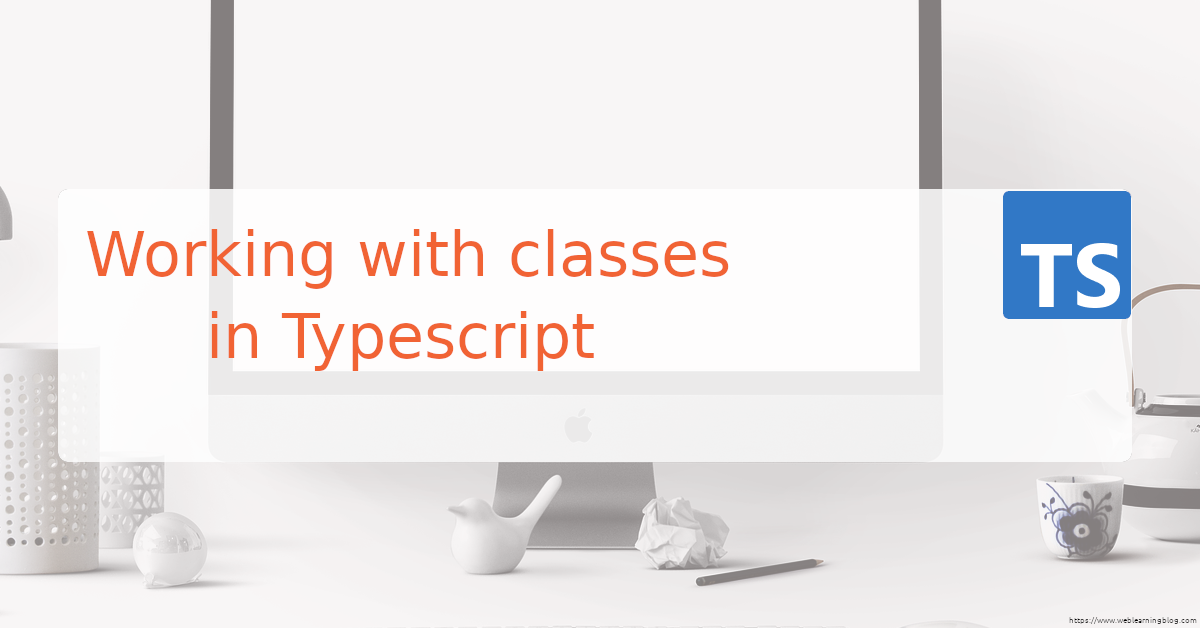
Working with classes in typescript Web Learning Blog
keyof typeof will infer the type of a javascript object and return a type that is the union of its keys. Because it can infer the exact value of the keys it can return a union of their literal types instead of just returning "string". type PreferenceKeys = keyof typeof preferences; // type '"language" | "theme"'.

Typescript Basics Part 2 Types, Classes and Inheritace TypeScript
Background and Syntax. In C#, we use many different types to represent collections, with various ways to create and initialize them. Many have verbose syntax, while some come with performance drawbacks, and together they make for a jumble of inconsistent code styles (ToList vs. ToArray, IEnumerable
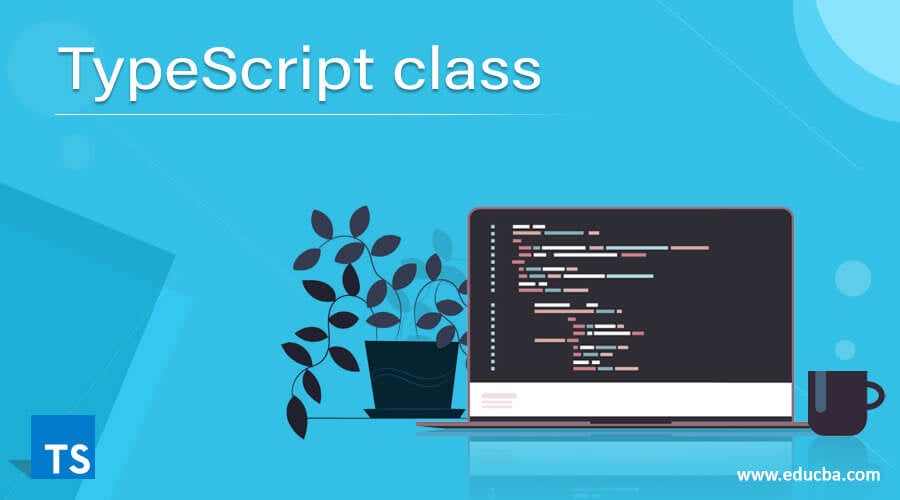
TypeScript class Working of classes in typescript with example and output
Interface Implemented by Class. In this approach, an interface named Shape is defined with a method calFn() that calculates a shape's area. The Rectangle class implements the Shape interface by providing its implementation of calFn(), the calculation of a rectangle's area when an instance of Rectangle is created and calFn() is called on that instance.

What is object and How to Declare object of a class in TypeScript?
18 Types for classes as values. 18.1 Types for specific classes. 18.2 The type operator typeof. 18.2.1 Constructor type literals. 18.2.2 Object type literals with construct signatures. 18.3 A generic type for classes: Class

TypeScript Core Concepts Using classes in TypeScript YouTube
You could add the following code to make the function type-safe in TypeScript: function identity
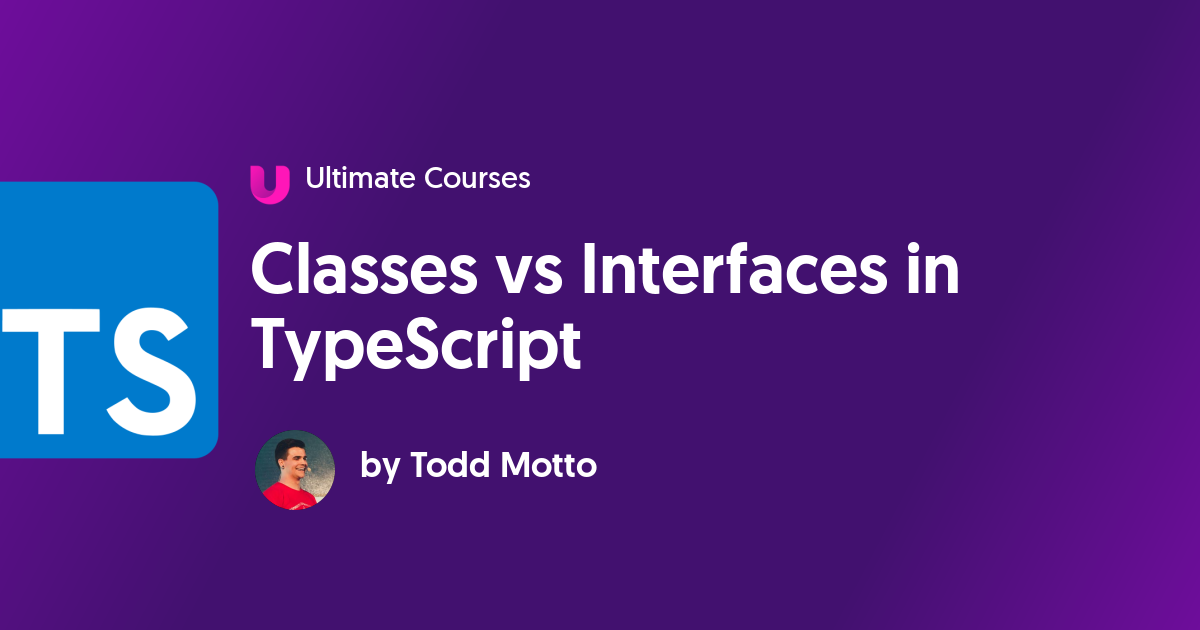
Classes vs Interfaces in TypeScript Ultimate Courses
The `typeof` operator in TypeScript is used for capturing the type of variables, providing a way to reuse the type information of existing structures. When used in type contexts, TypeScript's `typeof` can derive complex types from existing objects or variables, enhancing type safety. Type guards can be implemented effectively using `typeof.

Using an interface as a class in typescript by ERBO Engineering Mar
The typeof operator in TypeScript is incredibly versatile, allowing you to create types that are firmly anchored to the runtime values of your variables and properties. It promotes type safety and consistency throughout your codebase, ensuring that your types evolve along with your data. So, as you progress in your TypeScript journey, remember.
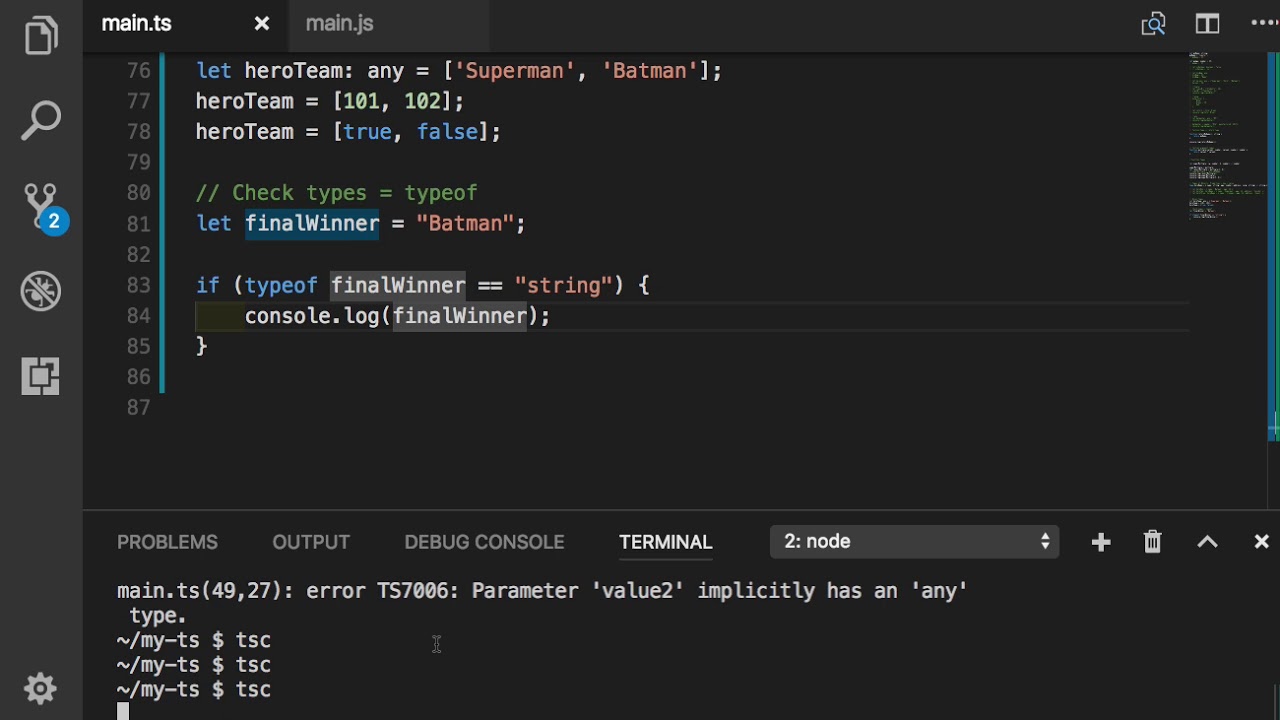
TypeScript 015 typeof YouTube
以下に、in演算子、instanceof演算子、typeof演算子、ユーザー定義の型ガード関数を使用した型ガードの例を示します。 in演算子. in演算子は、プロパティがオブジェクトに存在するかどうかをチェックするために使用されます。